#!/usr/bin/python3
import pymongo
import time
import datetime
import random
import threading
import prettytable as pt
myclient = pymongo.MongoClient("mongodb://10.10.10.150:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
tb = pt.PrettyTable()
tb.field_names = ["Records", "Insert Times"]
class myThread(threading.Thread):
def __init__(self, threadID, device_id, N):
threading.Thread.__init__(self)
self.threadID = threadID
self.device_id = device_id
self.N = N
def run(self):
print("开始线程:" + self.threadID)
checkMongo(self.threadID, self.device_id, self.N)
print("退出线程:" + self.threadID)
def checkMongo(threadID, device_id, N=10000):
''' 测试MongoDB插入速度 '''
start = time.time()
for i in range(N):
mydict = {
"time": datetime.datetime.now(),
"device_id": device_id,
"type": "virbation",
"value": random.randint(1, 100000000)
}
x = mycol.insert_one(mydict)
# print('Thread {} Insert record {} with returned ID: {}'.format threadID, i, x.inserted_id))
# time.sleep(3)
end = time.time()
print('Thread {}: Insert {} records with {} seconds.'.format(
threadID, N, end - start))
tb.add_row([N, end - start])
# 创建新线程
thread1 = myThread('1', "3126", 10000)
thread2 = myThread('2', "2117", 20000)
thread3 = myThread('3', "2109", 30000)
thread4 = myThread('4', "1107", 40000)
thread5 = myThread('5', "3122", 50000)
thread6 = myThread('6', "8101", 60000)
thread7 = myThread('7', "8102", 70000)
thread8 = myThread('8', "8103", 80000)
thread9 = myThread('9', "8104", 90000)
thread10 = myThread('10', "8120", 100000)
# 开启新线程
thread1.start()
thread2.start()
thread3.start()
thread4.start()
thread5.start()
thread6.start()
thread7.start()
thread8.start()
thread9.start()
thread10.start()
thread1.join()
thread2.join()
thread3.join()
thread4.join()
thread5.join()
thread6.join()
thread7.join()
thread8.join()
thread9.join()
thread10.join()
print(tb)
print("退出主线程")
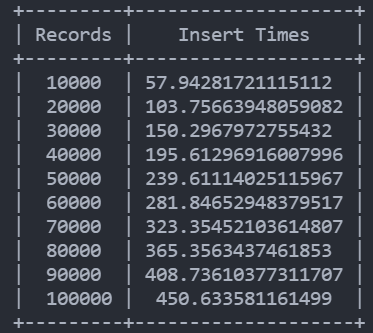